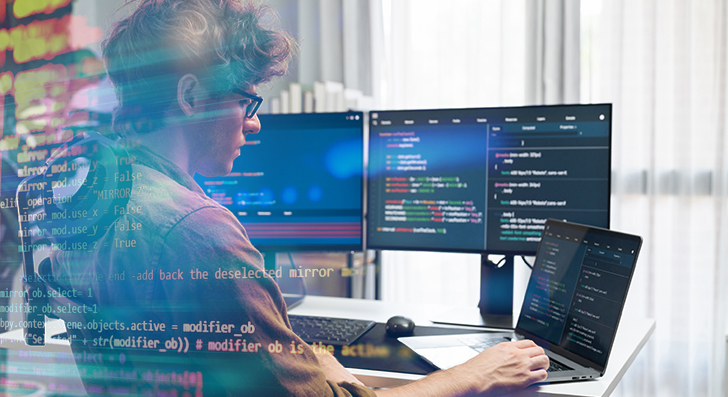
Scalability means your application can manage growth—a lot more users, extra knowledge, and a lot more site visitors—with out breaking. To be a developer, constructing with scalability in mind saves time and worry later on. Here’s a transparent and sensible guideline that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the beginning
Scalability isn't really something you bolt on later on—it ought to be portion of your prepare from the beginning. A lot of programs are unsuccessful after they mature quickly because the initial structure can’t manage the additional load. As being a developer, you need to Consider early regarding how your method will behave stressed.
Start by developing your architecture to generally be flexible. Prevent monolithic codebases in which all the things is tightly connected. Alternatively, use modular structure or microservices. These patterns split your application into lesser, independent elements. Every module or provider can scale By itself without affecting The entire process.
Also, give thought to your database from day one particular. Will it need to deal with 1,000,000 people or simply just a hundred? Choose the correct variety—relational or NoSQL—based upon how your details will expand. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional essential position is to stop hardcoding assumptions. Don’t produce code that only is effective below existing situations. Think of what would come about If the consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use design and style designs that help scaling, like message queues or occasion-driven systems. These help your application tackle additional requests without getting overloaded.
When you Establish with scalability in your mind, you are not just getting ready for success—you might be lessening long run complications. A very well-prepared program is easier to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the ideal Databases
Deciding on the appropriate database is actually a vital Component of making scalable apps. Not all databases are constructed the same, and utilizing the Mistaken you can sluggish you down or even induce failures as your application grows.
Begin by understanding your facts. Could it be highly structured, like rows inside of a desk? If Indeed, a relational database like PostgreSQL or MySQL is an effective fit. These are solid with associations, transactions, and consistency. In addition they help scaling techniques like read replicas, indexing, and partitioning to manage a lot more traffic and facts.
Should your details is much more adaptable—like user action logs, product catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured facts and can scale horizontally far more easily.
Also, take into account your read and produce patterns. Do you think you're accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you currently dealing with a significant write load? Explore databases that can manage significant write throughput, and even function-centered data storage methods like Apache Kafka (for short term facts streams).
It’s also good to Believe in advance. You might not need Sophisticated scaling functions now, but picking a databases that supports them suggests you received’t have to have to switch later.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your details depending on your access patterns. And always keep track of database overall performance as you develop.
In brief, the appropriate databases will depend on your application’s framework, pace wants, And the way you count on it to expand. Get time to pick wisely—it’ll conserve lots of difficulty later.
Improve Code and Queries
Speedy code is vital to scalability. As your app grows, each and every little delay provides up. Inadequately published code or unoptimized queries can decelerate efficiency and overload your method. That’s why it’s crucial to build economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Keep away from repeating logic and remove something unnecessary. Don’t pick the most intricate Answer if a straightforward one particular operates. Keep the features brief, concentrated, and simple to check. Use profiling applications to seek out bottlenecks—areas where by your code normally takes as well extensive to run or uses an excessive amount memory.
Up coming, evaluate your database queries. These normally gradual issues down much more than the code by itself. Be certain Every single question only asks for the information you truly require. Stay clear of Pick *, which fetches check here all the things, and as an alternative choose precise fields. Use indexes to speed up lookups. And keep away from doing too many joins, In particular across huge tables.
For those who recognize the exact same data currently being requested over and over, use caching. Retail outlet the results briefly working with tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database operations any time you can. Instead of updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more productive.
Make sure to exam with large datasets. Code and queries that function wonderful with one hundred data could possibly crash when they have to manage one million.
Briefly, scalable applications are speedy applications. Keep the code limited, your queries lean, and use caching when needed. These actions aid your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's to manage additional people plus more traffic. If everything goes through one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these tools assistance keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of a person server executing the many operate, the load balancer routes consumers to distinct servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can deliver traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-primarily based options from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info temporarily so it could be reused promptly. When end users request a similar data once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. You'll be able to provide it through the cache.
There are two common sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly obtain.
2. Shopper-side caching (like browser caching or CDN caching) outlets static information near the user.
Caching lowers database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve usually. And normally ensure your cache is current when information does adjust.
In short, load balancing and caching are basic but impressive resources. Together, they help your application tackle a lot more people, stay quickly, and Get well from problems. If you plan to increase, you would like each.
Use Cloud and Container Instruments
To make scalable applications, you will need tools that let your app increase conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling much smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t really need to obtain components or guess upcoming potential. When traffic increases, you are able to include a lot more sources with only a few clicks or instantly making use of automobile-scaling. When site visitors drops, it is possible to scale down to save cash.
These platforms also supply expert services like managed databases, storage, load balancing, and protection instruments. You may target constructing your app as opposed to handling infrastructure.
Containers are An additional key Software. A container offers your app and every little thing it must operate—code, libraries, configurations—into one particular unit. This makes it simple to maneuver your application among environments, from your notebook to your cloud, with no surprises. Docker is the most popular tool for this.
Once your app uses many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective application crashes, it restarts it instantly.
Containers also make it very easy to independent aspects of your app into services. You may update or scale elements independently, which is great for performance and dependability.
In short, working with cloud and container resources usually means you'll be able to scale speedy, deploy very easily, and Get better promptly when difficulties materialize. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, decrease possibility, and help you remain centered on building, not repairing.
Watch Every thing
In case you don’t observe your software, you received’t know when things go Improper. Checking allows you see how your app is doing, location issues early, and make much better selections as your application grows. It’s a vital part of creating scalable programs.
Get started by tracking fundamental metrics like CPU usage, memory, disk Room, and reaction time. These show you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this details.
Don’t just monitor your servers—keep track of your app as well. Keep watch over just how long it requires for end users to load web pages, how frequently problems come about, and the place they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a provider goes down, you ought to get notified right away. This assists you repair issues fast, normally in advance of people even observe.
Monitoring is also practical any time you make alterations. Should you deploy a brand new feature and see a spike in faults or slowdowns, it is possible to roll it back before it results in true harm.
As your application grows, targeted traffic and info increase. Devoid of monitoring, you’ll pass up indications of difficulty right until it’s way too late. But with the proper applications in position, you continue to be in control.
In short, checking helps you keep the app reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small apps have to have a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal resources, you may Develop applications that mature easily devoid of breaking under pressure. Start out small, Consider significant, and Develop clever.